Reactive Programming with JavaFX
#JavaFX
Java 8 is finally released and Lambda expression are an official part of Java. Thanks to this it’s much easier to write applications in a reactive way. One of the main concepts in reactive architecture is an event driven design. The reactive manifesto contains the following about event driven design:
In an event-driven application, the components interact with each other through the production and consumption of events—discrete pieces of information describing facts. These events are sent and received in an asynchronous and non-blocking fashion.
Reactive Programming with Java
By using Lambdas it’s very easy to define callbacks that can react on specific events. Here is a short example about an event driven design without the usage of Lambda expressions:
public static void hello(String... names) {
Observable.from(names).subscribe(new Action<String>() {
@Override
public void call(String s) {
System.out.println("Hello " + s + "!");
}
});
}
Thanks to Lambda expressions the same functionallity can be coded in Java 8 this way:
public static void hello(String... names) {
Observable.from(names).subscribe((s) -> System.out.println("Hello " + s + "!"));
}
The example is part of the RxJava tutorial.
Reactive Programming with JavaFX
Let’s take a look at JavaFX. Because the JavaFX API was designed for Java 8 it provides a lot of Lambda support and callbacks are used a lot. But next to the default JavaFX APIs there are currently an open source projects that adds a lot of reactive design and architecture to the JavaFX basics: ReactFX.
By using ReactFX you can do a lot of cool event driven stuff with only a few lines of code. Here is an example how event handlers can be designed to react on user inputs:
EventStream<MouseEvent> clicks = EventStreams.eventsOf(node, MouseEvent.MOUSE_CLICKED);
clicks.subscribe(click -> System.out.println("Click!"));
I think the API provides a lot of cool functionallity that let you design JavaFX applications that are more reactive and I hope to see a lot of more code like shown in the example above.
Summery
The are currently 2 cool reactive APIs for Java out there: RxJava for a basic use in Java and ReactFX that is specialized for JavaFX. Theoretically you can do everything (or most of the stuff) you can do with ReactFXwith the help of RxJava, too. But here you need to concern about the JavaFX Application Thread. Because ReactFX is implementated for JavaFX (or a single threaded environment) you don’t need to handle this. A first comparison of this two libraries can be found here.
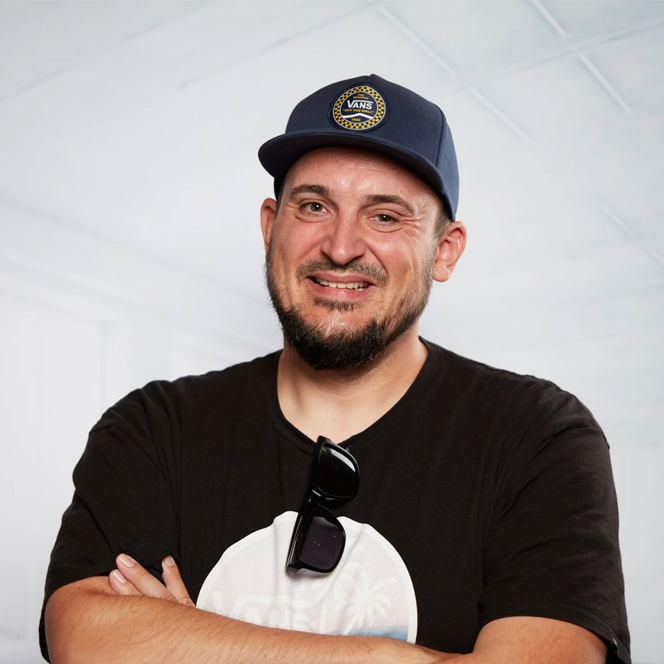
Hendrik Ebbers
Hendrik Ebbers is the founder of Open Elements. He is a Java champion, a member of JSR expert groups and a JavaOne rockstar. Hendrik is a member of the Eclipse JakartaEE working group (WG) and the Eclipse Adoptium WG. In addition, Hendrik Ebbers is a member of the Board of Directors of the Eclipse Foundation.